原理
说到Spring 的事务,大部分可能都会想到数据库对事务的支持所以才有了Spring 的事务机制,同时通过使用JDBC 的事务管理,那么你就得到了可以在Spring 中使用事务。
使用事务的示例:
1 2 3 4 5 6 7 8 9 10 11
| Connection conn = DriverManager.getConnection(); try { conn.setAutoCommit(false); 执行CRUD操作 conn.commit(); } catch (Exception e) { conn.rollback(); e.printStackTrace(); } finally { conn.colse(); }
|
从上面的示例中,我们就能看出来,事务是一系列的操作的集合,当集合中的某一部操作失败或者失误,那么在这之前的操作要全部回滚,并且终止后边的所有操作,避免出现由于数据不一致导致的一系列的错误。
事务的出现就是为了数据的完整性和一致性,目前在大部分的计算流程中,事务管理都是必不可少的。
基础
事务四大特性
事务四大特性(ACID):
- 原子性(Atomicity): 事务是一个原子操作,由一系列动作组成。事务的原子性确保动作要么全部完成,要么完全不起作用。
- 一致性(Consistency): 事务在完成时,必须是所有的数据都保持一致状态。
- 隔离性(Isolation): 并发事务执行之间无影响,在一个事务内部的操作对其他事务是不产生影响,这需要事务隔离级别来指定隔离性。
- 持久性(Durability): 一旦事务完成,数据库的改变必须是持久化的。
事务隔离级别
在日常应用的操作时,多用户并发使用数据库进行操作,那么在事务的并发中会导致以下的问题:
- 脏读: 一个事务读取到另一个事务的数据。
- 不可重复读: 一个事务读取同一行数据,两次读取获得的结果不一致。
- 幻读: 一个事务执行两次查询操作,两次获得数据不一致。
- 丢失更新: 当事务失败时,会将其他事务提交的数据覆盖。
那么为了解决事务并发存在的这些问题,就需要使用事务的隔离进行区分了。
- TRANSACTION_NONE JDBC: 驱动不支持事务
- TRANSACTION_READ_UNCOMMITTED: 允许脏读、不可重复读和幻读。
- TRANSACTION_READ_COMMITTED: 禁止脏读,但允许不可重复读和幻读。
- TRANSACTION_REPEATABLE_READ: 禁止脏读和不可重复读,单运行幻读。
- TRANSACTION_SERIALIZABLE: 禁止脏读、不可重复读和幻读。
上面的这些事务隔离,已经可以解决事务并发时导致的问题,但是为了解决上述的问题,那么在事务并发的性能方面就会被减弱,能处理的并发操作就要减少,所以需要根据具体的项目需求合理规划事物的隔离级别。
Spring 事务传播属性
Spring 共定义了7个以PROPAGATION_ 开头的常量来表示他的传播属性。
名称 |
值 |
解释 |
PROPAGATION_REQUIRED |
0 |
支持当前事务,如果当前没有事务,就新建一个事务。这是最常见的选择,也是Spring默认的事务的传播。 |
PROPAGATION_SUPPORTS |
1 |
支持当前事务,如果当前没有事务,就以非事务方式执行。 |
PROPAGATION_MANDATORY |
2 |
支持当前事务,如果当前没有事务,就抛出异常。 |
PROPAGATION_REQUIRES_NEW |
3 |
新建事务,如果当前存在事务,把当前事务挂起。 |
PROPAGATION_NOT_SUPPORTED |
4 |
以非事务方式执行操作,如果当前存在事务,就把当前事务挂起。 |
PROPAGATION_NEVER |
5 |
以非事务方式执行,如果当前存在事务,则抛出异常。 |
PROPAGATION_NESTED |
6 |
如果当前存在事务,则在嵌套事务内执行。如果当前没有事务,则进行与PROPAGATION_REQUIRED类似的操作。 |
Spring 事务隔离级别
名称 |
值 |
解释 |
ISOLATION_DEFAULT |
-1 |
这是一个PlatfromTransactionManager默认的隔离级别,使用数据库默认的事务隔离级别。另外四个与JDBC的隔离级别相对应。 |
ISOLATION_READ_UNCOMMITTED |
1 |
这是事务最低的隔离级别,它充许另外一个事务可以看到这个事务未提交的数据。这种隔离级别会产生脏读,不可重复读和幻读。 |
ISOLATION_READ_COMMITTED |
2 |
保证一个事务修改的数据提交后才能被另外一个事务读取。另外一个事务不能读取该事务未提交的数据。 |
ISOLATION_REPEATABLE_READ |
4 |
这种事务隔离级别可以防止脏读,不可重复读。但是可能出现幻读。 |
ISOLATION_SERIALIZABLE |
8 |
这是花费最高代价但是最可靠的事务隔离级别。事务被处理为顺序执行。除了防止脏读,不可重复读外,还避免了幻读。 |
Spring 事务管理
Spring 中事务管理的核心接口就是PlatformTransactionManager 。
1 2 3 4 5
| Public interface PlatformTransactionManager { TransactionStatus getTransaction(TransactionDefinition definition) throws TransactionException; void commit(TransactionStatus status) throws TransactionException; void rollback(TransactionStatus status) throws TransactionException; }
|
事务管理器接口通过getTransaction(TransactionDefinition definition) 方法根据指定的传播行为返回当前活动的事务或创建一个新的事务,这个方法里面的参数是TransactionDefinition 类,这个类就定义了一些基本的事务属性。并且返回值是一个TransactionStatus 接口的实现类,TransactionStatus 接口提供了控制事务执行和查询事务状态的方法。
在TransactionDefinition接口中定义了它自己的传播行为和隔离级别
1 2 3 4 5 6 7
| public interface TransactionDefinition { int getIsolationLevel(); String getName(); int getPropagationBehavior(); int getTimeout(); boolean isReadOnly(); }
|
1 2 3 4 5 6 7 8
| public interface TransactionStatus { void flush(); boolean hasSavepoint(); boolean isCompleted(); boolean isNewTransaction(); boolean isRollbackOnly(); void setRollbackOnly(); }
|
Spring 事务传播行为
事务传播行为是Spring 框架独有的事务增强特性,他并不属于的事务实际提供方数据库的行为。这是Spring 为我们提供的强大的工具,使用事务传播行可以为我们的开发工作提供许多便利。
而事务传播行为通俗解释就是用来描述由某一个事务传播行为修饰的方法被嵌套进另一个方法时的事务如何传播。
伪代码示例:
1 2 3 4 5 6 7 8 9
| public void methodA() { methodB(); } @Transaction(Propagation=XXX) public void methodB() { }
|
代码中methodA() 方法嵌套调用了methodB() 方法,而methodB() 的事务传播行为由@Transaction(Propagation=XXX) 设置决定。这里需要注意的是methodA() 并没有开启事务,所以某一个事务传播行为修饰的方法并不是必须要在开启事务的外围方法中调用。
Spring 中事务传播行为共分为7类:
事务传播行为类型 |
说明 |
PROPAGATION_REQUIRED |
如果当前没有事务,就新建一个事务,如果已经存在一个事务中,加入到这个事务中。这是最常见的选择。 |
PROPAGATION_SUPPORTS |
支持当前事务,如果当前没有事务,就以非事务方式执行。 |
PROPAGATION_MANDATORY |
使用当前的事务,如果当前没有事务,就抛出异常。 |
PROPAGATION_REQUIRES_NEW |
新建事务,如果当前存在事务,把当前事务挂起。 |
PROPAGATION_NOT_SUPPORTED |
以非事务方式执行操作,如果当前存在事务,就把当前事务挂起。 |
PROPAGATION_NEVER |
以非事务方式执行,如果当前存在事务,则抛出异常。 |
PROPAGATION_NESTED |
如果当前存在事务,则在嵌套事务内执行。如果当前没有事务,则执行与PROPAGATION_REQUIRED类似的操作。 |
事务回滚规则
在通常情况下,如果在事务中抛出了未检查异常(通常继承自 RuntimeException 的异常),那么则默认将回滚事务。如果没有抛出任何异常,或者抛出了已检查异常,则仍然提交事务。这通常也是大多数开发者希望的处理方式,也是 EJB 中的默认处理方式。但是,我们也可以根据需要人为地控制事务在抛出某些未检查异常时任然提交事务,或者在抛出某些已检查异常时回滚事务。
配置事务
编程式事务管理
在 Spring 出现以前,编程式事务管理对基于 POJO 的应用来说是唯一选择。 在Spring 出现之后,Spring 提供了对编程式事务和声明式事务的支持,编程式事务允许用户在代码中精确定义事务的边界,而声明式事务(基于AOP )有助于用户将操作与事务规则进行解耦。
TransactionTemplate
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| TransactionTemplate tt = new TransactionTemplate(); Object result = tt.execute( new TransactionCallback(){ public Object doTransaction(TransactionStatus status) { updateOperation(); return resultOfUpdateOperation(); } }); ````
### PlatformTransactionManager ```java DataSourceTransactionManager dataSourceTransactionManager = new DataSourceTransactionManager(); dataSourceTransactionManager.setDataSource(this.getJdbcTemplate().getDataSource()); DefaultTransactionDefinition transDef = new DefaultTransactionDefinition(); transDef.setPropagationBehavior(DefaultTransactionDefinition.PROPAGATION_REQUIRED); TransactionStatus status = dataSourceTransactionManager.getTransaction(transDef); try { dataSourceTransactionManager.commit(status); } catch (Exception e) { dataSourceTransactionManager.rollback(status); }
|
声明式事务管理
Spring 的声明式事务管理在底层是建立在 AOP 的基础之上的。其本质是对方法前后进行拦截,然后在目标方法开始之前创建或者加入一个事务,在执行完目标方法之后根据执行情况提交或者回滚事务。
声明式事务最大的优点就是不需要通过编程的方式管理事务,这样就不需要在业务逻辑代码中掺杂事务管理的代码,只需在配置文件中做相关的事务规则声明(或通过等价的基于标注的方式),便可以将事务规则应用到业务逻辑中。因为事务管理本身就是一个典型的横切逻辑,正是 AOP 的用武之地。Spring 开发团队也意识到了这一点,为声明式事务提供了简单而强大的支持。
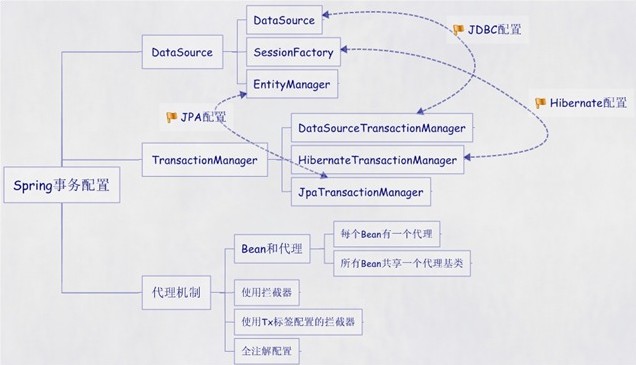
Spring配置文件中关于事务配置总是由三个组成部分,分别是DataSource、TransactionManager和代理机制这三部分,无论哪种配置方式,一般变化的只是代理机制这部分。
每个Bean都有一个代理
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-2.5.xsd">
<bean id="sessionFactory" class="org.springframework.orm.hibernate3.LocalSessionFactoryBean"> <property name="configLocation" value="classpath:hibernate.cfg.xml" /> <property name="configurationClass" value="org.hibernate.cfg.AnnotationConfiguration" /> </bean>
<bean id="transactionManager" class="org.springframework.orm.hibernate3.HibernateTransactionManager"> <property name="sessionFactory" ref="sessionFactory" /> </bean>
<bean id="userDaoTarget" class="com.bluesky.spring.dao.UserDaoImpl"> <property name="sessionFactory" ref="sessionFactory" /> </bean>
<bean id="userDao" class="org.springframework.transaction.interceptor.TransactionProxyFactoryBean"> <property name="transactionManager" ref="transactionManager" /> <property name="target" ref="userDaoTarget" /> <property name="proxyInterfaces" value="com.bluesky.spring.dao.GeneratorDao" /> <property name="transactionAttributes"> <props> <prop key="*">PROPAGATION_REQUIRED</prop> </props> </property> </bean> </beans>
|
所有Bean共享一个代理基类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-2.5.xsd">
<bean id="sessionFactory" class="org.springframework.orm.hibernate3.LocalSessionFactoryBean"> <property name="configLocation" value="classpath:hibernate.cfg.xml" /> <property name="configurationClass" value="org.hibernate.cfg.AnnotationConfiguration" /> </bean>
<bean id="transactionManager" class="org.springframework.orm.hibernate3.HibernateTransactionManager"> <property name="sessionFactory" ref="sessionFactory" /> </bean> <bean id="transactionBase" class="org.springframework.transaction.interceptor.TransactionProxyFactoryBean" lazy-init="true" abstract="true"> <property name="transactionManager" ref="transactionManager" /> <property name="transactionAttributes"> <props> <prop key="*">PROPAGATION_REQUIRED</prop> </props> </property> </bean> <bean id="userDaoTarget" class="com.bluesky.spring.dao.UserDaoImpl"> <property name="sessionFactory" ref="sessionFactory" /> </bean> <bean id="userDao" parent="transactionBase" > <property name="target" ref="userDaoTarget" /> </bean> </beans>
|
使用拦截器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-2.5.xsd">
<bean id="sessionFactory" class="org.springframework.orm.hibernate3.LocalSessionFactoryBean"> <property name="configLocation" value="classpath:hibernate.cfg.xml" /> <property name="configurationClass" value="org.hibernate.cfg.AnnotationConfiguration" /> </bean>
<bean id="transactionManager" class="org.springframework.orm.hibernate3.HibernateTransactionManager"> <property name="sessionFactory" ref="sessionFactory" /> </bean> <bean id="transactionInterceptor" class="org.springframework.transaction.interceptor.TransactionInterceptor"> <property name="transactionManager" ref="transactionManager" /> <property name="transactionAttributes"> <props> <prop key="*">PROPAGATION_REQUIRED</prop> </props> </property> </bean> <bean class="org.springframework.aop.framework.autoproxy.BeanNameAutoProxyCreator"> <property name="beanNames"> <list> <value>*Dao</value> </list> </property> <property name="interceptorNames"> <list> <value>transactionInterceptor</value> </list> </property> </bean> <bean id="userDao" class="com.bluesky.spring.dao.UserDaoImpl"> <property name="sessionFactory" ref="sessionFactory" /> </bean> </beans>
|
使用tx标签配置的拦截器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-2.5.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-2.5.xsd">
<context:annotation-config /> <context:component-scan base-package="com.bluesky" />
<bean id="sessionFactory" class="org.springframework.orm.hibernate3.LocalSessionFactoryBean"> <property name="configLocation" value="classpath:hibernate.cfg.xml" /> <property name="configurationClass" value="org.hibernate.cfg.AnnotationConfiguration" /> </bean>
<bean id="transactionManager" class="org.springframework.orm.hibernate3.HibernateTransactionManager"> <property name="sessionFactory" ref="sessionFactory" /> </bean>
<tx:advice id="txAdvice" transaction-manager="transactionManager"> <tx:attributes> <tx:method name="*" propagation="REQUIRED" /> </tx:attributes> </tx:advice> <aop:config> <aop:pointcut id="interceptorPointCuts" expression="execution(* com.bluesky.spring.dao.*.*(..))" /> <aop:advisor advice-ref="txAdvice" pointcut-ref="interceptorPointCuts" /> </aop:config> </beans>
|
全注解
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-2.5.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-2.5.xsd">
<context:annotation-config /> <context:component-scan base-package="com.bluesky" />
<tx:annotation-driven transaction-manager="transactionManager"/>
<bean id="sessionFactory" class="org.springframework.orm.hibernate3.LocalSessionFactoryBean"> <property name="configLocation" value="classpath:hibernate.cfg.xml" /> <property name="configurationClass" value="org.hibernate.cfg.AnnotationConfiguration" /> </bean>
<bean id="transactionManager" class="org.springframework.orm.hibernate3.HibernateTransactionManager"> <property name="sessionFactory" ref="sessionFactory" /> </bean> </beans>
|
在DAO上需加上@Transactional注解
后记
引用
https://segmentfault.com/a/1190000013341344 (详细介绍事务传播行为)
https://docs.spring.io/spring/docs/2.5.x/reference/index.html (官方文档)
总结
Spring 的出现宛如一块璞玉,你必须经过打磨才能看见其中的美玉。看来得找找时间看看Spring 的官方文档。
还是得要坚持天天学习啊,不然总感觉自己啥都不会。
个人备注
此博客内容均为作者学习所做笔记,侵删!
若转作其他用途,请注明来源!